String Length Problem: HSC Solution
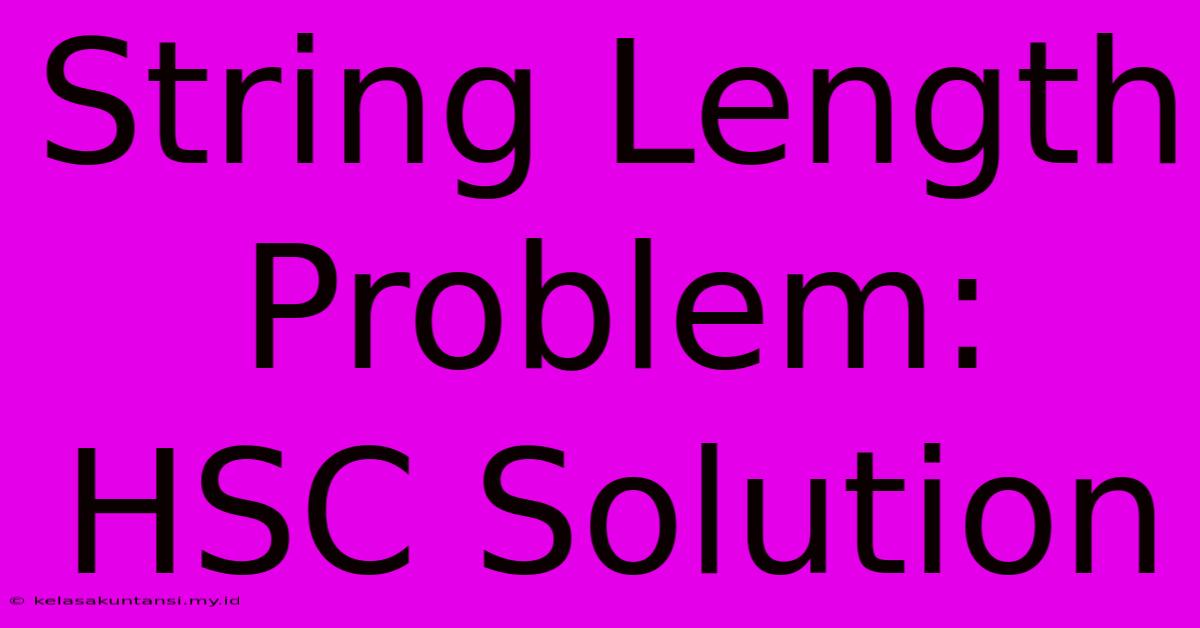
Temukan informasi yang lebih rinci dan menarik di situs web kami. Klik tautan di bawah ini untuk memulai informasi lanjutan: Visit Best Website meltwatermedia.ca. Jangan lewatkan!
Table of Contents
String Length Problem: HSC Solution
Determining the length of a string is a fundamental task in computer programming. This article provides a comprehensive guide to solving the string length problem, specifically tailored for Higher Secondary Certificate (HSC) level students. We'll explore various approaches, focusing on efficiency and clarity, essential for achieving high marks in your exams.
Understanding the Problem
The "string length problem" simply asks: how many characters are in a given string? This seemingly simple question has several ways to be tackled, each with its own advantages and disadvantages. Understanding the underlying data structures and algorithms is key to choosing the best solution. For HSC level, you'll likely be working with common programming languages like C++, Java, or Python.
Different Approaches to Solving the String Length Problem
Method 1: Using Built-in Functions
Most programming languages provide built-in functions designed specifically for this purpose. This is generally the most efficient and recommended approach for HSC level.
- C++: The
length()
method of thestring
class directly returns the string length. For example:
#include
#include
int main() {
std::string myString = "Hello, World!";
int len = myString.length();
std::cout << "String length: " << len << std::endl;
return 0;
}
- Java: The
length()
method of theString
class serves the same purpose.
public class StringLength {
public static void main(String[] args) {
String myString = "Hello, World!";
int len = myString.length();
System.out.println("String length: " + len);
}
}
- Python: The
len()
function is used to determine the length of a string.
myString = "Hello, World!"
string_length = len(myString)
print("String length:", string_length)
These built-in functions are optimized for performance and are the preferred method for most applications.
Method 2: Iterative Approach (For Understanding)
While less efficient than built-in functions, implementing a loop to count characters helps illustrate the underlying concept. This method is beneficial for understanding how string length is determined.
#include
#include
int main() {
std::string myString = "Hello, World!";
int len = 0;
for (char c : myString) {
len++;
}
std::cout << "String length: " << len << std::endl;
return 0;
}
This code iterates through each character in the string, incrementing a counter. This approach demonstrates the fundamental concept but is generally slower than using built-in functions.
Choosing the Right Method
For your HSC exams, prioritizing readability and efficiency is crucial. Using the built-in functions (Method 1) is strongly recommended. Understanding the iterative approach (Method 2) is valuable for grasping the underlying principles, but for practical applications and exam performance, stick to the optimized built-in solutions.
Common Mistakes to Avoid
- Off-by-one errors: Carefully consider the starting and ending points of your loops if implementing an iterative solution.
- Incorrect function usage: Ensure you are using the correct built-in function for your chosen programming language.
- Handling null or empty strings: Your code should gracefully handle cases where the input string is empty or
NULL
.
Q&A
Q: Can I use recursion to find the string length?
A: While technically possible, recursion is generally inefficient for this task and not recommended. Iterative approaches or built-in functions are far superior in terms of performance.
Q: What happens if my string contains special characters?
A: Built-in functions handle special characters without any issues. They count each character, regardless of its type.
Conclusion
Determining string length is a fundamental programming concept. Mastering this skill is essential for HSC students. By understanding the various approaches and choosing the most efficient method (the built-in functions), you can ensure your code is both correct and optimized for performance. Remember to practice consistently and focus on clear, well-documented code. Good luck with your exams!
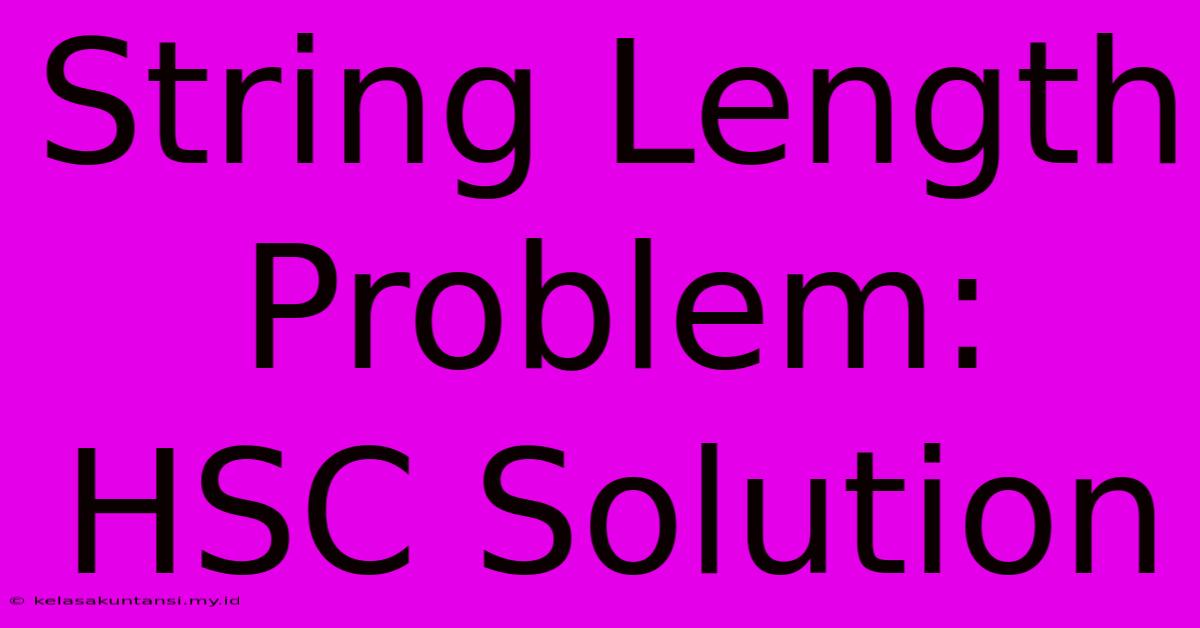
Football Match Schedule
Upcoming Matches
Latest Posts
Terimakasih telah mengunjungi situs web kami String Length Problem: HSC Solution. Kami berharap informasi yang kami sampaikan dapat membantu Anda. Jangan sungkan untuk menghubungi kami jika ada pertanyaan atau butuh bantuan tambahan. Sampai bertemu di lain waktu, dan jangan lupa untuk menyimpan halaman ini!
Kami berterima kasih atas kunjungan Anda untuk melihat lebih jauh. String Length Problem: HSC Solution. Informasikan kepada kami jika Anda memerlukan bantuan tambahan. Tandai situs ini dan pastikan untuk kembali lagi segera!
Featured Posts
-
Vanuatu Earthquake Garage Cctv Footage
Dec 18, 2024
-
Facundo Molesta A Faloon Y Anyella Llora
Dec 18, 2024
-
Academy Awards 10 Categories Shortlisted
Dec 18, 2024
-
En Vivo Psg Vs Monaco Pacho Juega
Dec 18, 2024
-
Zweden Succesvol Zalmbeschermingsproject
Dec 18, 2024