Measuring String Length: HSC Insights
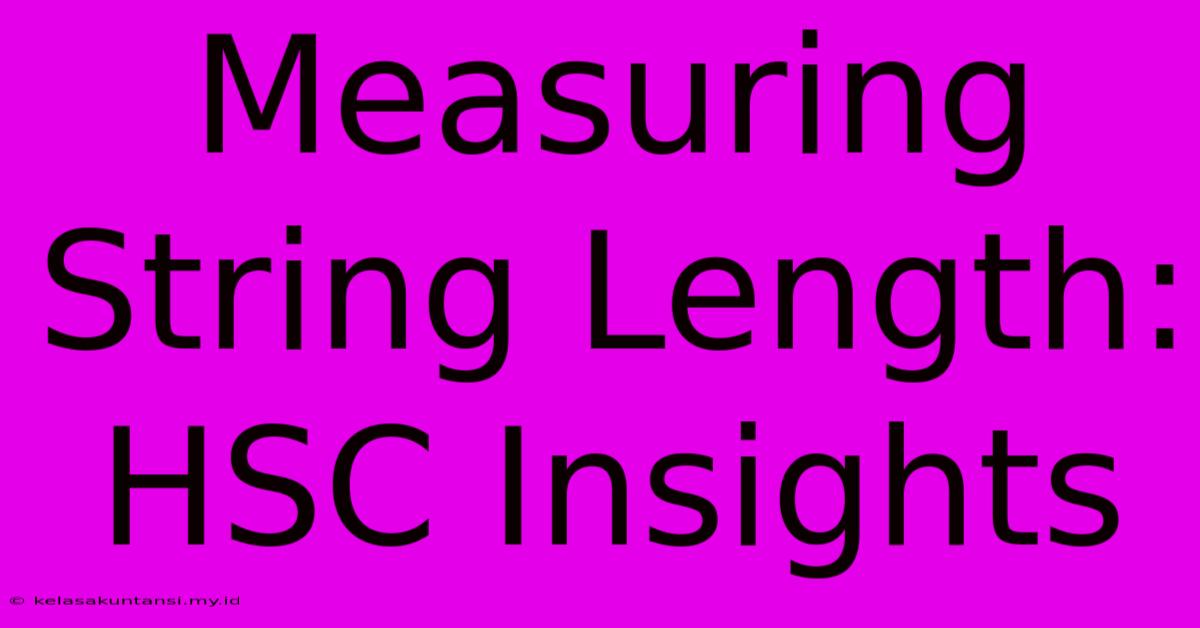
Temukan informasi yang lebih rinci dan menarik di situs web kami. Klik tautan di bawah ini untuk memulai informasi lanjutan: Visit Best Website meltwatermedia.ca. Jangan lewatkan!
Table of Contents
Measuring String Length: HSC Insights
Determining the length of a string is a fundamental task in many programming scenarios. This article delves into efficient methods for measuring string length, specifically focusing on insights relevant to Higher School Certificate (HSC) Computer Science students. We'll explore various approaches and their implications, helping you master this crucial concept.
Understanding String Length
Before diving into methods, let's establish a clear understanding. String length refers to the number of characters within a string. This includes letters, numbers, spaces, and special characters. Empty strings have a length of zero.
Why is Measuring String Length Important?
Measuring string length is vital for various programming tasks, including:
- Data validation: Ensuring input fields meet specific length requirements.
- String manipulation: Performing operations like substring extraction or padding.
- Memory management: Estimating memory allocation needs for string storage.
- Algorithm design: Many algorithms rely on string length for efficiency.
Methods for Measuring String Length
Different programming languages offer various ways to determine string length. We will focus on common approaches applicable across many languages.
Using Built-in Functions
Most programming languages provide a built-in function specifically designed for this purpose. For example, in Python, we use len()
. In Java, it's length()
. These functions are generally highly optimized for performance.
my_string = "Hello, HSC!"
string_length = len(my_string)
print(f"The length of the string is: {string_length}")
This Python example demonstrates how simple it is to obtain the string length using the built-in len()
function. The output will be "The length of the string is: 12".
Manual Iteration (Less Efficient)
While less efficient than built-in functions, manually iterating through the string character by character can offer educational value. This method enhances understanding of the underlying string structure.
my_string = "HSC Programming"
count = 0
for char in my_string:
count += 1
print(f"The length of the string is: {count}")
This Python code illustrates manual iteration. It's less efficient but helps solidify the concept of string traversal.
Optimizing String Length Measurement
For most applications, using the built-in function is the best approach. It's optimized and generally faster than manual methods. However, understanding the underlying principles remains crucial for problem-solving.
HSC Exam Relevance
Understanding string length measurement is crucial for various HSC Computer Science topics. Questions might involve string manipulation, algorithm design, or data validation, all requiring a solid grasp of string length. Practicing various approaches will enhance your problem-solving capabilities.
Q&A
Q: What happens if I try to measure the length of a null string?
A: The length of a null or empty string will always be 0. Built-in functions handle this gracefully.
Q: Are there any performance differences between the built-in function and manual iteration?
A: Yes, the built-in function is significantly faster and more efficient, particularly for longer strings. Manual iteration has a time complexity directly proportional to string length.
Q: Can I measure string length in characters with special symbols?
A: Yes. The length function counts all characters including spaces, punctuation, and special characters.
Conclusion
Measuring string length is a seemingly simple yet fundamental concept in programming. Mastering this skill, along with understanding different approaches, is essential for success in HSC Computer Science and beyond. Focusing on efficiency through built-in functions while understanding the underlying principles will solidify your understanding and prepare you for more complex programming challenges. Remember to practice regularly to improve your proficiency!
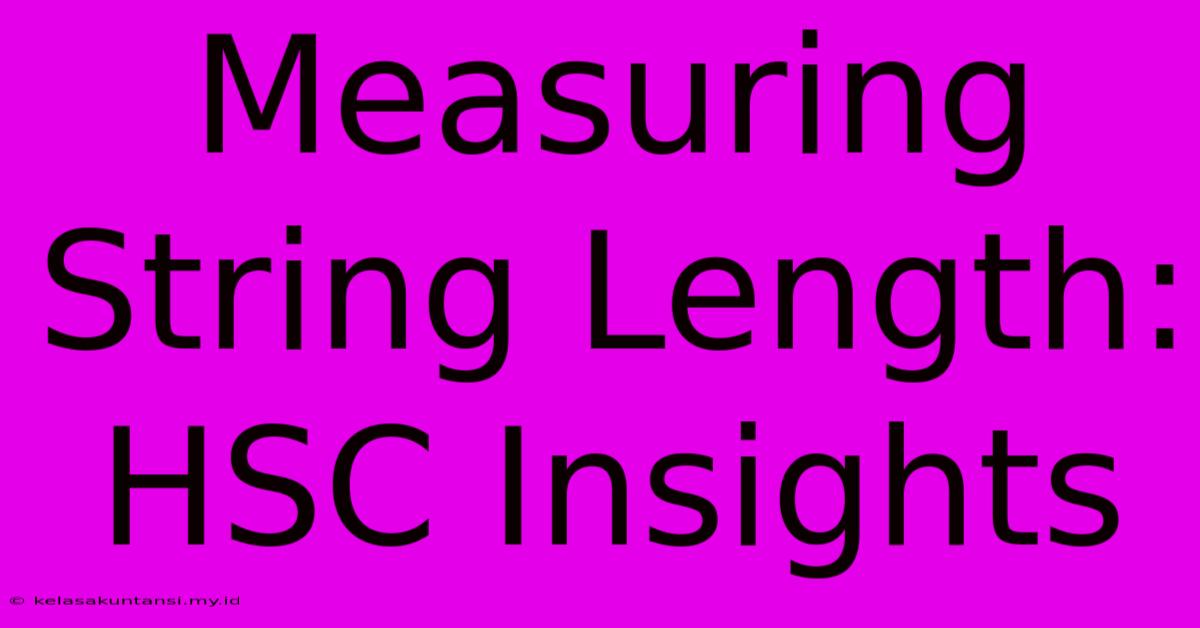
Football Match Schedule
Upcoming Matches
Latest Posts
- How to Improve Your SEO Skills
Published on: 2024-12-01 - Understanding the Basics of HTML5
Published on: 2024-11-30 - Tips Learn Trading for Beginners
Published on: 2024-11-28
Terimakasih telah mengunjungi situs web kami Measuring String Length: HSC Insights. Kami berharap informasi yang kami sampaikan dapat membantu Anda. Jangan sungkan untuk menghubungi kami jika ada pertanyaan atau butuh bantuan tambahan. Sampai bertemu di lain waktu, dan jangan lupa untuk menyimpan halaman ini!
Kami berterima kasih atas kunjungan Anda untuk melihat lebih jauh. Measuring String Length: HSC Insights. Informasikan kepada kami jika Anda memerlukan bantuan tambahan. Tandai situs ini dan pastikan untuk kembali lagi segera!
Featured Posts
-
Trump Nominiert Feinberg Fuer Luxemburg
Dec 18, 2024
-
Los Mas Escuchados Spotify Colombia 2024
Dec 18, 2024
-
Four Wet Years Fuel Bushfire Concerns
Dec 18, 2024
-
Fallon Sherrock Darts And Nierenkrankheit
Dec 18, 2024
-
Allscripts Analysis Big Data Healthcare Market
Dec 18, 2024